mirror of
https://github.com/chefyuan/algorithm-base.git
synced 2024-12-27 12:56:17 +00:00
添加py和js,添加注释
This commit is contained in:
parent
813cfddd0b
commit
4a8c81e88e
@ -4,7 +4,7 @@
|
||||
>
|
||||
> 另外希望手机阅读的同学可以来我的 <u>[**公众号:袁厨的算法小屋**](https://raw.githubusercontent.com/tan45du/test/master/微信图片_20210320152235.2pthdebvh1c0.png)</u> 两个平台同步,想要和题友一起刷题,互相监督的同学,可以在我的小屋点击<u>[**刷题小队**](https://raw.githubusercontent.com/tan45du/test/master/微信图片_20210320152235.2pthdebvh1c0.png)</u>进入。
|
||||
|
||||
有的老哥说链表的排序搞不明白,让我写一下,一不小心给忘记了,今天咱们就安排上。没有学过数据结构的同学可以先看下这个文章
|
||||
有的老哥说链表的排序搞不明白,让我写一下,一不小心给忘记了,今天咱们就安排上。没有学过数据结构的同学可以先看下这个文章:
|
||||
|
||||
[【绘图解析】链表详解](https://github.com/chefyuan/algorithm-base/blob/main/animation-simulation/%E6%95%B0%E6%8D%AE%E7%BB%93%E6%9E%84%E5%92%8C%E7%AE%97%E6%B3%95/%E5%85%B3%E4%BA%8E%E9%93%BE%E8%A1%A8%E7%9A%84%E9%82%A3%E4%BA%9B%E4%BA%8B.md)
|
||||
|
||||
@ -32,13 +32,11 @@
|
||||
|
||||
我们知道链表查询元素的时间复杂度为 O(n),我们只能够通过遍历链表查询元素。
|
||||
|
||||
那么我们怎么才能将新元素放到合适的位置呢?
|
||||
|
||||
**见下图**
|
||||
那么我们怎么才能将新元素放到合适的位置呢?见下图。
|
||||
|
||||
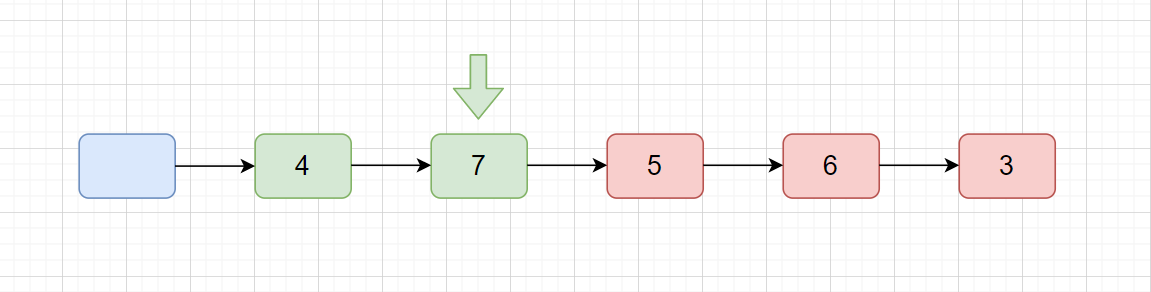
|
||||
|
||||
此时我们不能通过移动绿色指针来寻找 5 的合适位置,那么我们应该怎么做呢?见下图
|
||||
此时我们不能通过移动绿色指针来寻找 5 的合适位置,那么我们应该怎么做呢?见下图。
|
||||
|
||||
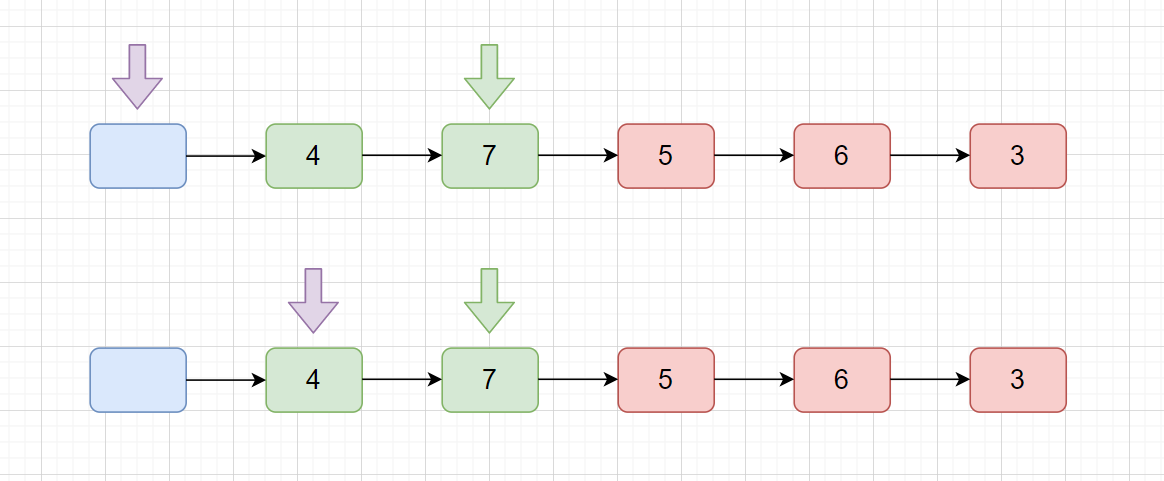
|
||||
|
||||
@ -54,13 +52,13 @@
|
||||
|
||||
下面我们再来看动画模拟具体过程。
|
||||
|
||||
**注:为了更好的表达算法思想,让过程更流畅,特省略了指针的移动细节,直接插入到合适位置,后面会详细说明插入操作的具体步骤**
|
||||
**注:为了更好的表达算法思想,让过程更流畅,特省略了指针的移动细节,直接插入到合适位置,后面会详细说明插入操作的具体步骤。**
|
||||
|
||||
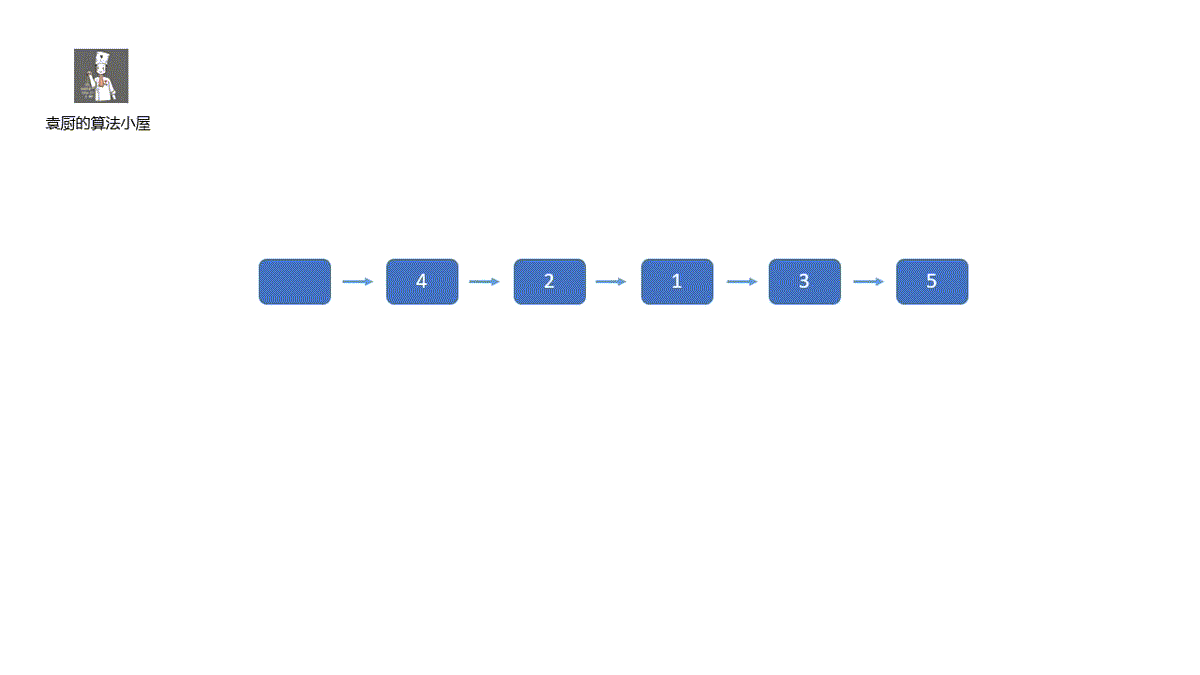
|
||||
|
||||
我们通过上面的动画知道了大致过程,那么我们的是如何将新元素插入到指定位置的呢?
|
||||
|
||||
见下图
|
||||
见下图。
|
||||
|
||||
|
||||
|
||||
@ -68,7 +66,7 @@
|
||||
|
||||
|
||||
|
||||
我们想要将 3 插入到 2 和 4 的中间,此时我们三个指针分别指向 2,4,3
|
||||
我们想要将 3 插入到 2 和 4 的中间,此时我们三个指针分别指向 2,4,3。
|
||||
|
||||
我们共分 4 步,来完成这个操作,见下图
|
||||
|
||||
@ -87,7 +85,6 @@ Java Code:
|
||||
```java
|
||||
class Solution {
|
||||
public ListNode insertionSortList(ListNode head) {
|
||||
|
||||
if (head == null && head.next == null) {
|
||||
return head;
|
||||
}
|
||||
@ -98,7 +95,6 @@ class Solution {
|
||||
//判断是否需要执行插入操作
|
||||
ListNode pre = head.next;
|
||||
ListNode last = head;
|
||||
ListNode temphead = dummyNode;
|
||||
while (pre != null) {
|
||||
//不需要插入到合适位置,则继续往下移动
|
||||
if (last.val <= pre.val) {
|
||||
@ -107,7 +103,7 @@ class Solution {
|
||||
continue;
|
||||
}
|
||||
//开始出发,查找新元素的合适位置
|
||||
temphead = dummyNode;
|
||||
ListNode temphead = dummyNode;
|
||||
while (temphead.next.val <= pre.val) {
|
||||
temphead = temphead.next;
|
||||
}
|
||||
@ -115,10 +111,10 @@ class Solution {
|
||||
last.next = pre.next;
|
||||
pre.next = temphead.next;
|
||||
temphead.next = pre;
|
||||
//继续往下移动
|
||||
pre = last.next;
|
||||
}
|
||||
return dummyNode.next;
|
||||
|
||||
}
|
||||
}
|
||||
```
|
||||
@ -139,7 +135,6 @@ public:
|
||||
//判断是否需要执行插入操作
|
||||
ListNode * pre = head->next;
|
||||
ListNode * last = head;
|
||||
ListNode * temphead = dummyNode;
|
||||
while (pre != nullptr) {
|
||||
//不需要插入到合适位置,则继续往下移动
|
||||
if (last->val <= pre->val) {
|
||||
@ -150,12 +145,13 @@ public:
|
||||
//开始出发,查找新元素的合适位置
|
||||
temphead = dummyNode;
|
||||
while (temphead->next->val <= pre->val) {
|
||||
temphead = temphead->next;
|
||||
ListNode * temphead = temphead->next;
|
||||
}
|
||||
//此时我们已经找到了合适位置,我们需要进行插入,大家可以画一画
|
||||
last->next = pre->next;
|
||||
pre->next = temphead->next;
|
||||
temphead->next = pre;
|
||||
//继续往下移动
|
||||
pre = last->next;
|
||||
}
|
||||
return dummyNode->next;
|
||||
@ -163,5 +159,71 @@ public:
|
||||
};
|
||||
```
|
||||
|
||||
JS Code:
|
||||
|
||||
```javascript
|
||||
var insertionSortList = function(head) {
|
||||
if (head === null || head.next === null) return head;
|
||||
//哑节点
|
||||
let dummyNode = new ListNode(-1, head);
|
||||
let pre = head.next;
|
||||
//pre负责指向新元素,last 负责指向新元素的前一元素
|
||||
//判断是否需要执行插入操作
|
||||
let last = head;
|
||||
while (pre) {
|
||||
//不需要插入到合适位置,则继续往下移动
|
||||
if (last.val <= pre.val) {
|
||||
last = last.next;
|
||||
pre = pre.next;
|
||||
continue;
|
||||
}
|
||||
//开始出发,查找新元素的合适位置
|
||||
let tempHead = dummyNode;
|
||||
while (tempHead.next.val <= pre.val) {
|
||||
tempHead = tempHead.next;
|
||||
}
|
||||
//此时我们已经找到了合适位置,我们需要进行插入,大家可以画一画
|
||||
last.next = pre.next;
|
||||
pre.next = tempHead.next;
|
||||
tempHead.next = pre;
|
||||
//继续往下移动
|
||||
pre = last.next;
|
||||
}
|
||||
return dummyNode.next;
|
||||
};
|
||||
```
|
||||
|
||||
Python Code:
|
||||
|
||||
```py
|
||||
class Solution:
|
||||
def insertionSortList(self, head: ListNode) -> ListNode:
|
||||
if head is None or head.next is None:
|
||||
return head
|
||||
# 哑节点
|
||||
dummyNode = ListNode(-1, head)
|
||||
# pre负责指向新元素,last 负责指向新元素的前一元素
|
||||
# 判断是否需要执行插入操作
|
||||
pre = head.next
|
||||
last = head
|
||||
while pre is not None:
|
||||
# 不需要插入到合适位置,则继续往下移动
|
||||
if last.val <= pre.val:
|
||||
pre = pre.next
|
||||
last = last.next
|
||||
continue
|
||||
# 开始出发,查找新元素的合适位置
|
||||
temphead = dummyNode
|
||||
while temphead.next.val <= pre.val:
|
||||
temphead = temphead.next
|
||||
# 此时我们已经找到了合适位置,我们需要进行插入,大家可以画一画
|
||||
last.next = pre.next
|
||||
pre.next = temphead.next
|
||||
temphead.next = pre
|
||||
# 继续往下移动
|
||||
pre = last.next
|
||||
return dummyNode.next
|
||||
```
|
||||
|
||||
|
||||
|
||||
|
Loading…
Reference in New Issue
Block a user